Hey Engineers. Merry Christmas and we’re back with a new tutorial. We will discuss how to play Christmas songs using Arduino Uno and a piezoelectric buzzer. We used a small piezoelectric buzzer to play simple Jingle bells using Arduino Uno. We have made the code such that it’s already set melody and timing so that when PWM pulses vary HIGH-LOW Buzzer will produce a perfectly timed PWM output to the Buzzer. So below are the complete components with circuits and code and Video that explain how we can play jingle bells using Arduino.
Materials
- Arduino Uno – 1Pc
- 10R resistor -1Pc
- RED-LED -1Pc
- Piezo Buzzer (Small)
- Bread Board (any type)
- 5V DC Power supply
Circuit Diagram for our Jingle Bells Using Arduino Project
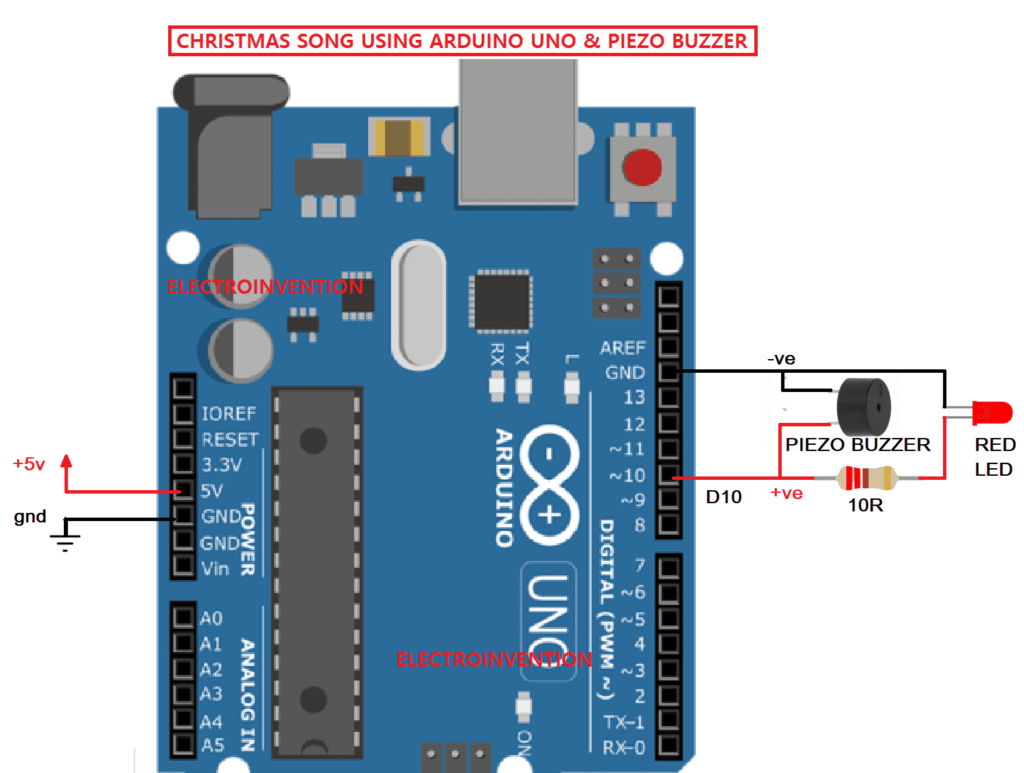
Arduino with Buzzer Circuit Interface
In this circuit above, we made it a lot simple and also added an LED to tune in with the buzzer song. As in the circuit above, the whole setup can be powered by a 5 Volts DC Power Supply.
You can also power it through your PC USB Port which is also 5v DC. You can use any Arduino board that you’re having according to pin configuration. We need PWM(Pulsed Width Modulation) output in order to make a jingle bell melody using Arduino.
With the Arduino Uno, we are using here, we have PINs 3, 5, 6, 9, 10, and 11 as PWM PINs. For output, we have used PWM PIN 10 as the output to the speaker.
Let’s Move to the Arduino Code and Video Below.
Arduino code for playing Jingle Bells using Buzzer
//defining the notes with freq. Code looks much similar to one on internet, but its much more optimized //and tested for better sound quality diagram and code at: https://electroinvention.co.in
#define C 2100
#define D 1870
#define E 1670
#define f 1580
#define G 1400
// defining special note, 'R', as a rest
#define R 0
// Use PWM PINs for the Speaker output (We use D10 here )
int speakerOut = 10;
void setup() {
pinMode(speakerOut, OUTPUT);
}
// melody and time delay code
// melody[] is an array of notes, followed by the beats[],
// which sets each note's relative length (higher #, longer note)
int melody[] = { E, E, E, R,
E, E, E, R,
E, G, C, D, E, R,
f, f, f, f, f, E, E, E, E, D, D, E, D, R, G, R,
E, E, E, R,
E, E, E, R,
E, G, C, D, E, R,
f, f, f, f, f, E, E, E, G, G, f, D, C, R };
int MAX_COUNT = sizeof(melody) / 2; // using C language sizeof operator for setting melody length
long tempo = 8000; // this delay in ms for length of pause between the notes
int pause = 100;
// Loop variable to increase Rest length
int rest_count = 100;
// Initializing the core variables
int tone_ = 0;
int beat = 0;
long duration = 0;
//playing the jingle bell tune
// Pulse generation for the speaker to play a tone for a set time period
void playTone() {
long elapsed_time = 0;
if (tone_ > 0) { // if no rest, while the tone has
// played less long than 'duration', pulse speaker HIGH and LOW
while (elapsed_time < duration) {
digitalWrite(speakerOut, HIGH);
delayMicroseconds(tone_ / 3);
// DOWN
digitalWrite(speakerOut, LOW);
delayMicroseconds(tone_ / 3);
// Keep track of how long we pulsed
elapsed_time += (tone_);
}
} else { // Rest beat; loop times delay
for (int j = 0; j < rest_count; j++) { // See NOTE on rest_count
delayMicroseconds(duration);
}
}
}
void loop() {
for (int i = 0; i < MAX_COUNT; i++) {
tone_ = melody[i];
beat = 60; //beat to 60
duration = beat * tempo; //setting up timing
playTone();
// adding delay pause between notes
delayMicroseconds(pause);
}
}
To play Christmas Song using Arduino we need to make the code a perfectly timed melody with an array of notes and set frequency timing between low and high pulses.
With the code above, you can see the description of each and every line in the comments, with “//” written along each Line of Code. The code is edited and optimized for better sound quality to give you more precise and sweet melody music.
Video
We hope you enjoyed it, Stay safe, and merry Christmas. If you liked our Content, do subscribe to our newsletter, it’s Free!
Stay tuned to this site for the latest post updates, projects, Free Books, Quizzes, Giveaways, and much more. Subscribe to our Newsletter for Free and Comment Below.
you posted on after so much duration man. Nice post TBH
Thanks Bruna, Thanks for visiting